Looking Back at 20-year-old Code
Development#javascript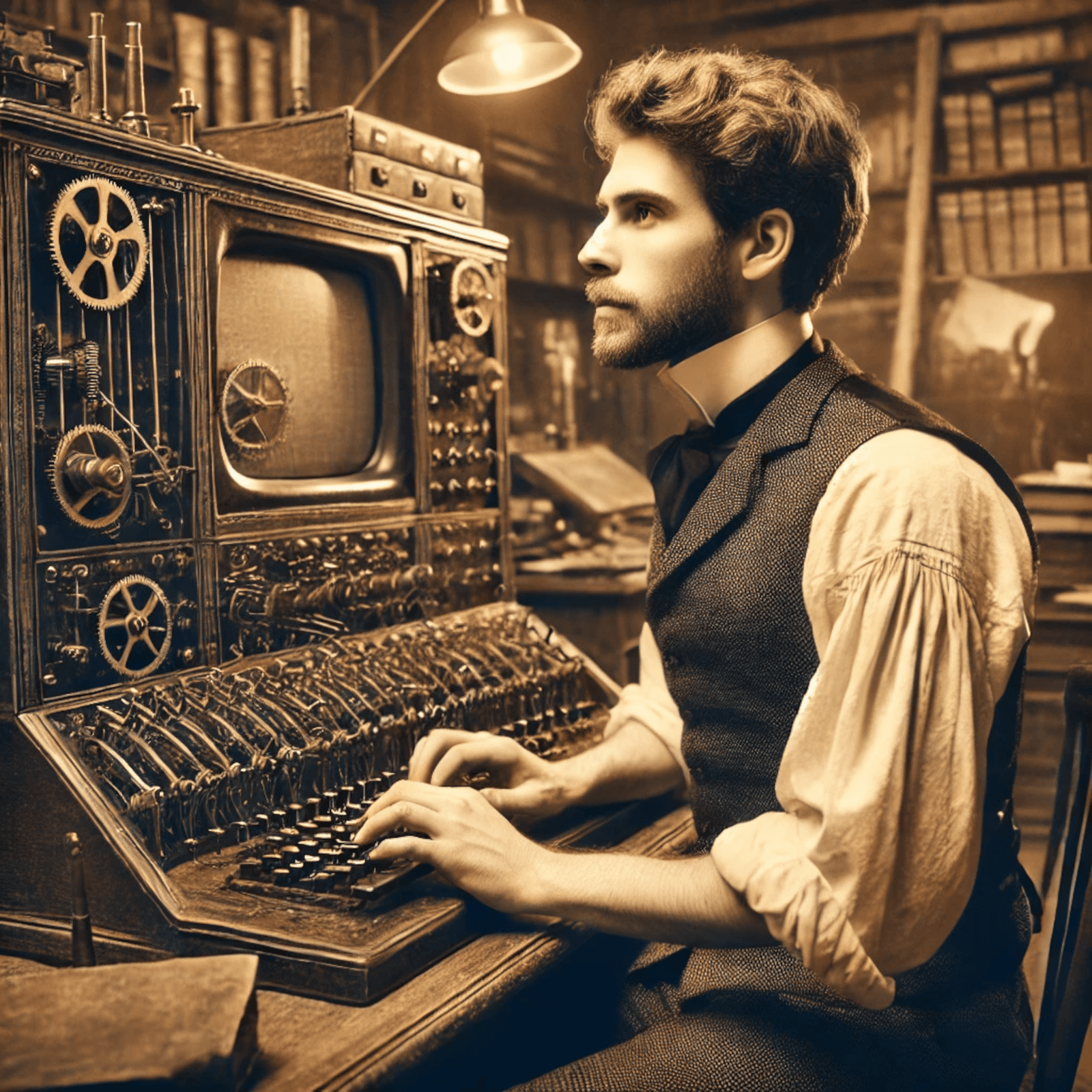
Introduction
If my Javascript calendar from 2001 on GitHub was a human being, she would be 20 years old and might be nearing the end of a degree course by now. It's one of my first ever scripts, and it is long overdue a code review. I thought I'd just go through it and pick out some interesting pieces.
Before I start, I should actually say that without me touching it at all the code from 20 years ago worked. It took a while to load because of the Google Analytics code failing to load I believe, but the calendar loaded eventually! I did tweak it slightly when I added it to GitHub in 2016.
Refactoring
Other than removing the Google Analytics code, the first thing I had to do was give myself a history lesson. Once upon a time there was a browser called Netscape that appears to have treated the year part of dates differently to other browsers...
var dateYear = todayDate.getYear()
if(navigator.appName.indexOf("Netscape") != -1){
dateYear = 1900 + parseInt(dateYear);
}
It looks like these days getYear()
has been replaced by getFullYear()
to overcome this issue...
let currentYear = todayDate.getFullYear()
The way I'm listing the arrays isn't too bad, but it's just very clunky...
var dateDayArray = new Array()
dateDayArray[0] = "Sunday"
dateDayArray[1] = "Monday"
dateDayArray[2] = "Tuesday"
dateDayArray[3] = "Wednesday"
dateDayArray[4] = "Thursday"
dateDayArray[5] = "Friday"
dateDayArray[6] = "Saturday"
It's worse that I'd actually copied and pasted the same code elsewhere on the page and changed the name of the second array.
These days, as well as using let
it's much quicker to write the same thing using square brackets, shorthand for an array, because we know what the indexes will be...
let dayArray = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"]
Not too bad so far, but after all those arrays we're down to line 70 already. The next part is the first part that makes me really cringe...
// get the day of the first of the month!!!
var dateDay = todayDate.getDay()
var dayInt = parseInt(dateDay);
var dateOneDay;
if ((dateDate == 1)||(dateDate == 8)||(dateDate == 15)||(dateDate == 22)||(dateDate == 29)){
dateOneDay = dayInt;
}else if ((dateDate == 2)||(dateDate == 9)||(dateDate == 16)||(dateDate == 23)||(dateDate == 30)){
dateOneDay = dayInt-1;
}else if ((dateDate == 3)||(dateDate == 10)||(dateDate == 17)||(dateDate == 24)||(dateDate == 31)){
dateOneDay = dayInt-2;
}else if ((dateDate == 4)||(dateDate == 11)||(dateDate == 18)||(dateDate == 25)){
dateOneDay = dayInt-3;
}else if ((dateDate == 5)||(dateDate == 12)||(dateDate == 19)||(dateDate == 26)){
dateOneDay = dayInt-4;
}else if ((dateDate == 6)||(dateDate == 13)||(dateDate == 20)||(dateDate == 27)){
dateOneDay = dayInt-5;
}else if ((dateDate == 7)||(dateDate == 14)||(dateDate == 21)||(dateDate == 28)){
dateOneDay = dayInt-6;
}
if (dateOneDay == -1){
dateOneDay = 6;
}else if (dateOneDay == -2){
dateOneDay = 5;
}else if (dateOneDay == -3){
dateOneDay = 4;
}else if (dateOneDay == -4){
dateOneDay = 3;
}else if (dateOneDay == -5){
dateOneDay = 2;
}else if (dateOneDay == -6){
dateOneDay = 1;
}
First of all, I'm not entirely sure why I've decided to start indenting all of a sudden. It was possibly nested inside something else before it was moved. I should say, by the way, I'm not sure what I wrote this on, but it could well have been notepad. Refactoring would have been a struggle, so I'll let myself off.
Next, I'm looking for the first day of the month, i.e. the first of the month will be on a ___day. I'm saying if today is the 1st, 8th, 15th, 22nd or 29th of the month, today must be the same day as the first of the month. It definitely gets the job done. Another thing that is confusing is the variable names, is it a day or a date. But, why am I re-using variables? Why??
Anyway, a better way to do this might be...
let todayDate = new Date()
let firstDayOfMonth = new Date(todayDate.getFullYear(), todayDate.getMonth(), 1);
let dateOneDay = firstDayOfMonth.getDay();
But, I've already assigned todayDate.getFullYear()
to a variable, so I could just re-use that.
The main for loop...
for(var i=0; i<42; i++){
if(i < dateOneDay){
num[i] = "<img src='images/blank.gif' border=0 width=50 height=50>";
} else if (i == dateOneDay){
currentDay = 1;
if (dateDate == 1){
num[i] = "<img src='images/today" + 1 + ".gif' border=0 width=50 height=50 alt='" + currentDay + "'>";
}else{
num[i] = "<img src='images/day" + 1 + ".gif' border=0 width=50 height=50 alt='" + currentDay + "'>";
}
} else if (currentDay+1 == dateDate){
currentDay += 1;
num[i] = "<img src='images/today" + currentDay + ".gif' border=0 width=50 height=50 alt='" + currentDay + "'>";
} else if (currentDay == numDays){
num[i] = "<img src='images/blank.gif' border=0 width=50 height=50>";
endNum = currentDay + dateOneDay;
} else if (currentDay > numDays){
num[i] = "<img src='images/blank.gif' border=0 width=50 height=50>";
} else {
currentDay += 1;
num[i] = "<img src='images/day" + currentDay + ".gif' border=0 width=50 height=50 alt='" + currentDay + "'>";
}
}
document.writeln("<DIV>");
document.writeln(num[0]);
document.writeln(num[1]);
I'm assigning each string to an array because I was to hardcode the DIV
tags in manually where I want them to be.
There is a lot of duplication and as such it's confusing to read.
Also, I don't like why I have to add one to the currentDay.
In fact, I'm defining currentDay in one of the else if
blocks.
The HTML attributes don't help with the readability, but it was written 20 years ago.
The Result
To be fair, this isn't hugely better, but it strips out some duplication and is slightly nicer to read.
It does avoid the need to hardcode each square with the DIV
tags.
I would have added the DIV
tags in 2016, originally this was a table, I'm sure.
function writeCalendarSquares() {
let thisDay = 1;
for (let i = 0; i < 42; i++) {
if (continueRows(i, thisDay) === false) {
break;
}
thisDay = writeDaySquare(i, thisDay);
endRow(i);
}
}
function writeDaySquare(i, thisDay) {
if (i < dateOneDay || thisDay > numDays) {
outputText = "<img src='images/blank.gif' alt=''>";
} else if (thisDay === currentDate) {
outputText = "<img src='images/today" + thisDay + ".gif' alt='" + thisDay + "'>";
thisDay++;
} else {
outputText = "<img src='images/day" + thisDay + ".gif' alt='" + thisDay + "'>";
thisDay++;
}
document.writeln(outputText);
return thisDay;
}
function continueRows(i, thisDay) {
if (i % 7 === 0) {
if (thisDay > numDays) {
return false;
}
document.writeln("<DIV>");
}
return true;
}
function endRow(i) {
if ((i + 1) % 7 === 0) {
document.writeln("</DIV>");
}
}
Conclusion for 2024
This was a completely pointless exercise and re-factoring old code might not be the best way to do this. A better way would probably be to start again from scratch for something very small, like this.
The original code was plain javascript with no framework, so my refactoring used the same approach, i.e. I did not use a framework. These days I'd say that any new project would tend to use a framework. There are pros and cons to anything. While using a framework in 2024 is probably more acceptable than not using a framework, there will also be a lot of dependencies with a framework. The chances of a project built with a framework just working after 20 years of not being touched are quite remote.
The javascript language itself, has changed in the 20 years, and browsers have also changed, as discussed. An exercise such as this just shows how few breaking changes there have been over a long timeframe when there is no framework. Different languages will have behaved differently over the past 20 years, but the general principal will be the same.
In reality, most projects are far bigger than this and starting over isn't generally an option. In this case, refactoring the code relatively quickly improved the code quite a lot.
A dilemma that a developer is more likely to have is whether to refactor or not. If it functions correctly, and is not affecting anything else, what are the reasons to refactor?
Updated 2024-08-19